Azure App Configuration: an introduction
By rickvdbosch
- 7 minutes read - 1315 wordsAzure App Configuration is a powerful way to manage and store application configuration on a central location. Integrating it into for instance ASP.NET (Core) is pretty straightforward. This post gives you an introduction to Azure App Configuration and a simple example of how to integrate it in ASP.NET Core.
Creating an Azure App Configuration 🆕
Of course there are several ways to create a specific resource in the Azure Portal. One of the simplest is to go to the portal, open up App Configuration and click the Add button. The form below will appear.
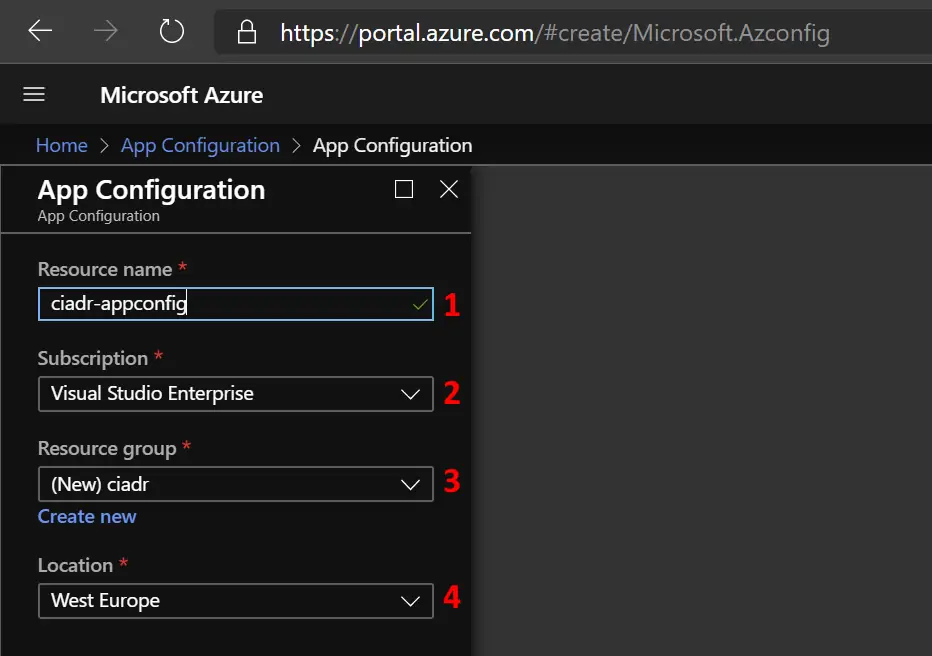
Creating an App Configuration in the Azure Portal
- The globally unique name of the App Configuration resource (this will be the prefix in the URL)
In this case, the URL will be https://ciadr-appconfig.azconfig.io - The subscription to create the App Configuration in
- The Resource Group to create the App Configuration in
- The location of the App Configuration
As soon as you have all information filled in, click the Create button. That’s it! You just created your (first?) Azure App Configuration.
Understanding the possibilities 🤯
There’s a decent amount of documentation on (the possibilities of) Azure App Configuration available over at Microsoft Docs.
Azure App Configuration provides a service to centrally manage application settings and feature flags. Modern programs, especially programs running in a cloud, generally have many components that are distributed in nature. Spreading configuration settings across these components can lead to hard-to-troubleshoot errors during an application deployment. Use App Configuration to store all the settings for your application and secure their accesses in one place.
What is Azure App Configuration?
The two most important things available in App Configuration are 1) Feature management and 2) Keys and Values.
Other features: Feature management đź’ˇ
Feature flags (or feature toggles or switches) are a way of determining which feature is available for which (group of) user(s). A decent feature management solution can help you manage quick changes to feature availability on demand. To implement feature flags, your application needs to make use of them, and you need a separate storage for the feature flags and their state. I’m planning for a separate post on implementing feature flags using Azure App Configuration.
If you’re interested in reading more about feature flags and the possibilities App Configuration has for it, take a look at the Feature management overview documentation.
Other features: Point-in-time snapshot ⏲
Azure App Configuration keeps records of the precise times when a new key-value pair is created and then modified. These records form a complete timeline in key-value changes. An App Configuration store can reconstruct the history of any key value and replay its past value at any given moment, up to the present. With this feature, you can “time-travel” backward and retrieve an old key value.
Point-in-time snapshot
Other features: Event handling ⚡
Azure App Configuration events enable applications to react to changes in key-values. This is done without the need for complicated code or expensive and inefficient polling services. Instead, events are pushed through Azure Event Grid to subscribers such as Azure Functions, Azure Logic Apps, or even to your own custom HTTP listener, and you only pay for what you use.
Reacting to Azure App Configuration events
Keys and Values đź—„
This introduction focuses on actual configuration in App Configuration. In the end, configuration of an application is (roughly) nothing more than some values you can request based on their keys. Think about the AppSettings element in a web.config file.
Keys and values in App Configuration give you exactly that. And more 🤓.
Configuration explorer
For managing keys and values, you go to the Configuration explorer. This immediately shows us there’s more than just keys and values…
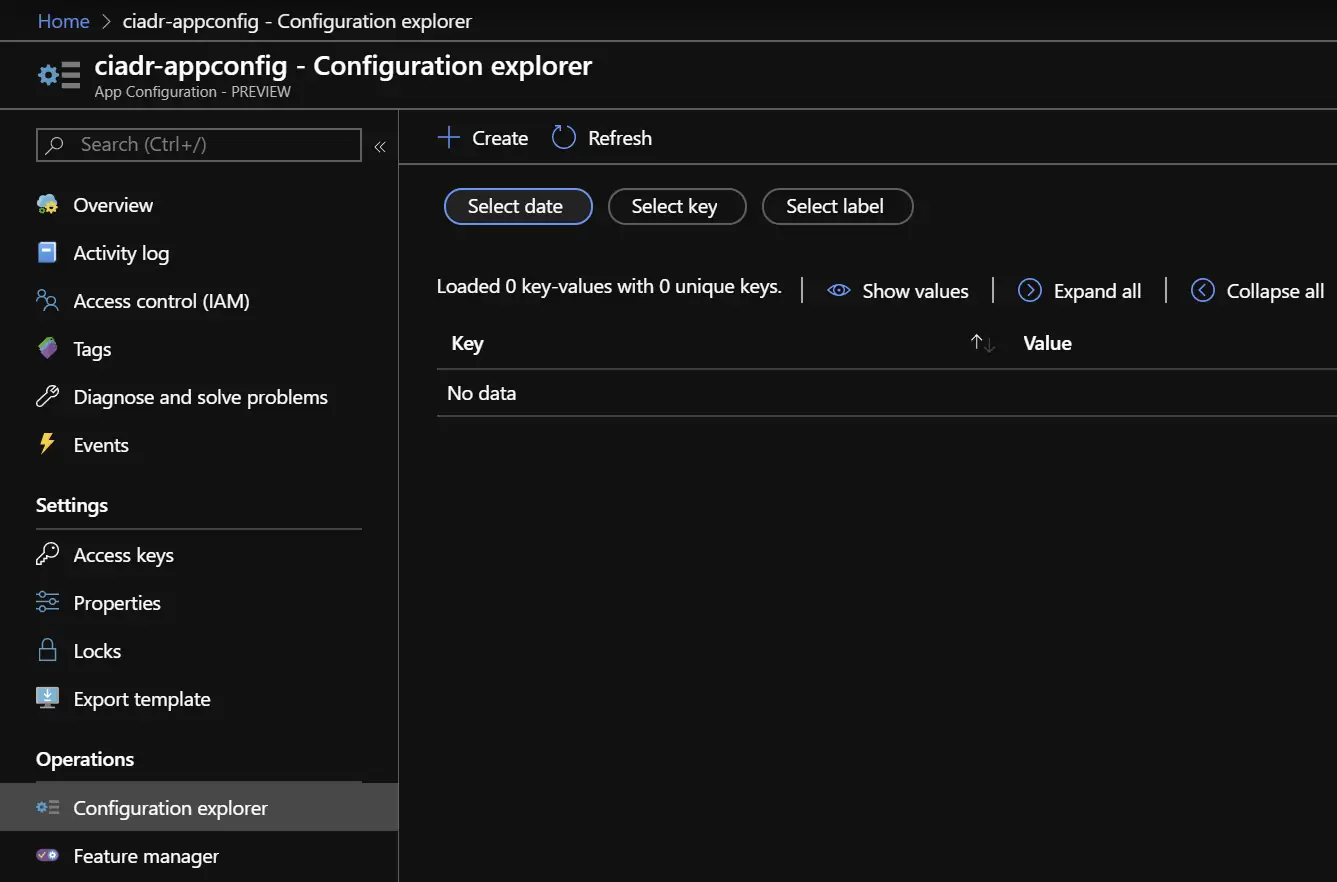
Configuration explorer
There are three different types of filters available:
The date filter đź“…
As mentioned in the chapter Point-in-time snapshot, App Configuration stores a complete timeline in key-value changes. By clicking the ‘Select date’ button, you get a date and time selector to specify the point-in-time you want to see the configuration for that exact moment.

Select date
The filter works instantly, and will show you all settings that were there at that specified moment, and the values they had then.
Filtering on keys 🔑
You can filter on keys, enabling you to for instance prefix keys for specific customers. There’s some decent documentation on how to Query key values.
In short:
Each key value is uniquely identified by its key plus a label that can be
null
. You query an App Configuration store for key values by specifying a pattern. The App Configuration store returns all key values that match the pattern and their corresponding values and attributes.
Filtering on labels 🏷
Labels are optional fields for a key-value pair, that can be used to differentiate key values with the same key. For instance, a key Setting1 with labels STG and PRD are actually two separate keys in an App Configuration store.
Create a new key-value pair
There’s a Create button there that let’s you create a new key-value-pair. If you click it, there’s another little surprise:
Next to adding a ’normal’ key-value pair, you can also add a ’ Key vault reference’. This will, together with a Managed Identity, let the platform help you getting a secret from Key Vault without you having to write any code.
So the UI for actually creating a new key-value looks like this:
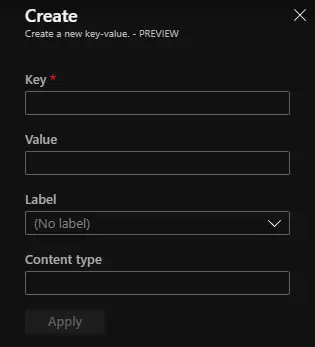
Create a new key-value
The Key and Value fields speak for themselves. We talked about Labels, too. The one thing to add here is that the Label dropdown will be populated with any labels that were already used in this App Configuration. The last field in this form is that of Content type. Providing a proper content-type can enable transformations of values when they are retrieved by applications.
Writing the code!
First, we add Azure App Configuration to the ConfigurationBuilder
when creating the IHostBuilder
.
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
webBuilder.ConfigureAppConfiguration((webHostBuilderContext, configurationBuilder) =>
{
// Comment 1.
var settings = configurationBuilder.Build();
configurationBuilder.AddAzureAppConfiguration(options =>
{
// Comment 2
options.Connect(settings["AppConfigurationConnectionString"])
.Select(KeyFilter.Any, null)
.Select(KeyFilter.Any, "Test")
.Select(KeyFilter.Any, "Acc")
.Select(KeyFilter.Any, "Prod");
});
});
});
}
Comments
- First we build the IConfigurationBuilder to be able to access settings in the sources already added (appsettings.json).
- If you don’t specify a label to filter on, you only get values with a null label. As soon as you specify a label, you only get values with that label. And then the extra-good news: you can stack filters! The last filter ‘wins’ (stacks over the rest). So this configuration roughly translates into:
- When there’s a setting with the label ‘Prod’, give me that one.
- If a value labeled ‘Prod’ is not there, but there is a value labeled ‘Acc’, give me that one.
- When there is no value labeled ‘Acc’, but there’s a value labeled ‘Test’, give me that one.
- If there’s no value with the label ‘Test’, give me the one without a label.
Next, to get values from App Configuration you simply inject IConfiguration
into for instance your Razor Page class and get settings from it like you would in any other scenario:
// Field for holding the IConfiguration
private readonly IConfiguration _configuration;
// Public property to bind against from the view
public string Setting1 { get; set; }
public IndexModel(IConfiguration configuration)
{
_configuration = configuration;
}
// Getting the value from the IConfiguration like you normally would.
// This gets the value from App Configuration (if it's in there)
public void OnGet()
{
Setting1 = _configuration.GetValue<string>(nameof(Setting1));
}
Resources
Video
I did a talk on this topic earlier this year, and I got the opportunity to talk about it in an episode of dotnetFlix. You can find the episode here:
Working example
For the code I used in this examples (and in the dotnetFlix video), see the GitHub repo rickvdbosch/ciadr.
Hope this helps! If you have any questions, don’t hesitate to contact me.